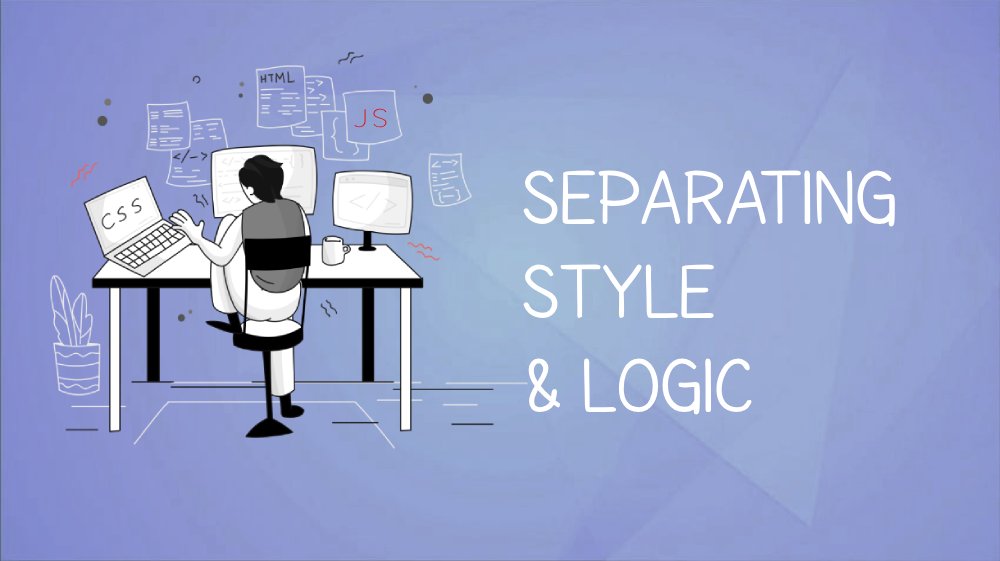
Introduction
In software development, the user interface (UI) acts as the interaction layer, facilitating communication between human users and the application’s core functionality. It’s the visual layer where users interact, shaping their experience and perception of the software. From sleek buttons to intuitive menus and dynamic forms, the UI plays a pivotal role in crafting seamless interactions.
At the heart of every UI lie its components – the fundamental building blocks that collectively construct the user experience. These components range from basic elements like buttons and text inputs to more complex entities such as grids, navigation bars, modals, and accordions. Each component serves a specific purpose, contributing to the overall functionality and aesthetic appeal of the UI.
Traditionally, UI components have been tightly coupled with their presentation logic, resulting in limited flexibility and reusability. However, a new paradigm known as headless UI components is changing the game. By decoupling the logic and presentation layers, headless UI components offer unparalleled flexibility and customization options for developers. This approach allows developers to wield these components like Lego blocks, assembling them in novel ways to craft tailored user experiences across various platforms and devices.
In this article, we’ll delve into the fascinating world of headless UI components, exploring their definition, features, use cases, and implementation strategies. Join us as we embark on a journey to unlock the full potential of headless UI components in modern web development.
Why headless UI components:
In the realm of UI development, headless components represent a paradigm shift in how we approach building user interfaces. Headless UI components represent a novel architectural approach in web development where the functionality and logic of a component are separated from its presentation or styling. In essence, these components focus solely on providing the underlying functionality without imposing any specific visual design. This separation allows developers to have more control and flexibility over the appearance and styling of the user interface.
Using an Analogy:
To better understand headless component architecture, let’s draw an analogy with a car. In this analogy, imagine the car engine as the headless component. The engine is responsible for providing the power and functionality necessary for the car to operate, such as propelling it forward and controlling speed. However, the engine does not dictate the exterior design or appearance of the car.
Similarly, in headless component architecture, the component acts as the engine, handling the underlying functionality and logic of an element within the user interface. This could include tasks such as handling user interactions, managing state, or performing data retrieval and manipulation. However, just like the car’s exterior design is independent of the engine, the styling of the UI component is left entirely up to the developer. They are free to apply their own styles, themes, or design system conventions to achieve the desired visual appearance.
In summary, headless UI components embody a modular and flexible approach to building user interfaces, where functionality and presentation concerns are decoupled. This architectural pattern empowers developers to create highly customizable and adaptable user interfaces, akin to designing a car with a powerful engine that can be paired with any exterior design.
Benefits of using headless UI components
Headless UI components offer several advantages that can significantly improve your development workflow and the end product. Let’s delve deeper into some of these key benefits:
1. Increased Flexibility:
One of the most significant advantages of headless UI components is their incredible flexibility. Unlike traditional UI components that come with predefined styles, headless components focus solely on functionality. This allows you to style them according to your existing design system, ensuring a cohesive and consistent user experience across your application. You’re not limited to the aesthetics provided by the library, but rather have complete control over the look and feel, from colors and fonts to layouts and animations. This empowers you to create a truly unique and brand-aligned user interface.
2. Improved Accessibility:
Many popular headless UI libraries come with built-in accessibility features. These features ensure that your components are usable by everyone, regardless of their abilities. This includes features like keyboard navigation, focus management, and screen reader support. By leveraging these pre-built features, you save development time while guaranteeing a more inclusive user experience. Headless UI libraries often prioritize accessibility best practices, making it easier to build applications that comply with accessibility standards.
3. Enhanced Reusability:
Headless UI components are designed to be modular and reusable. Thanks to their focus on core functionality, you can easily create custom components by combining and extending the basic building blocks. This allows you to build more complex UI elements from simpler ones, promoting code reuse and reducing development time. Additionally, reusable components can be easily shared within your team, promoting consistency and a streamlined development process.
4. Lighter Weight:
Headless UI components typically have a smaller bundle size compared to their styled counterparts. This is because they don’t carry the weight of the visual styles. This translates to faster loading times for your application, especially beneficial for mobile users or those with slower internet connections. A smaller bundle size also contributes to improved overall performance and a smoother user experience.
Potential drawbacks of headless UI components
While headless UI components offer numerous advantages, it’s important to consider some potential drawbacks before fully embracing this approach:
1. Increased Development Time:
Headless UI components require more development effort compared to pre-styled components. Since they lack built-in styles, developers need to handle the styling themselves, integrating them with your design system. This can add time to the development process, especially for projects with complex UIs. Additionally, some UX decisions related to styling and layout might need to be made from scratch, further contributing to development time.
2. Less Opinionated Approach:
Headless UI components are intentionally less opinionated in terms of design. They provide the functionality but leave the aesthetics entirely up to you. While this flexibility is a strength, it can also be challenging for some developers. The lack of pre-defined styles may require additional upfront planning and design system integration to ensure a cohesive look and feel. This can be particularly true for teams without a well-established design system.
3. Potential for Reinventing the Wheel:
The modularity of headless UI components can be a double-edged sword. While it allows for customization and building complex components, it also carries the risk of unintentionally recreating existing functionality. Developers might spend time building custom components that already exist within the headless UI library or elsewhere. To avoid this pitfall, it’s crucial to thoroughly explore the library’s offerings and existing community resources before diving into custom development.
Usage Example
In the following example, I demonstrate the usage of a headless button component in a React application. The HeadlessButton
component encapsulates the functionality of a button without specifying any specific visual design, adhering to the principles of headless UI components. We’ll explore how to integrate this component into a React application and customize its appearance using CSS at the integration level.
HeadlessButton.tsx (Headless button creation)
import React from 'react';
// Define the Props interface for the HeadlessButton component
interface HeadlessButtonProps {
onClick: () => void;
children: React.ReactNode;
className?: string; // Allow custom class names for further styling
}
// Headless Button Component
const HeadlessButton: React.FC<HeadlessButtonProps> = ({ onClick, children, className }) => {
const handleClick = () => {
if (onClick) {
onClick();
}
};
return (
<button className={`headless-button ${className}`} onClick={handleClick}>
{children}
</button>
);
};
export default HeadlessButton;
MyComponent.tsx (Usage example)
import React from 'react';
import './HeadlessButton.css'; // Import CSS for basic styling
import HeadlessButton from './HeadlessButton';
// Usage Example
const MyComponent: React.FC = () => {
const handleClick = () => {
console.log('Button clicked!');
};
return (
<div>
<h1>Headless Button Example</h1>
<p>Basic button:</p>
<HeadlessButton {...{ onClick: handleClick, children: 'Click me!' }} />
<p>Primary button:</p>
<HeadlessButton {...{ onClick: handleClick, children: 'Click me!', className: 'primary' }} />
</div>
);
};
export default MyComponent;
HeadlessButton.css (Basic styling at integration level)
.headless-button {
/* Basic styles */
padding: 8px 16px;
border: 1px solid #ccc;
background-color: #fff;
color: #333;
font-size: 14px;
cursor: pointer;
transition: background-color 0.3s, color 0.3s;
}
.headless-button:hover {
/* Hover styles */
background-color: #f0f0f0;
}
.headless-button.primary {
/* Custom primary button styles */
background-color: #007bff;
color: #fff;
border-color: #007bff;
}
.headless-button.primary:hover {
/* Hover styles for primary button */
background-color: #0056b3;
border-color: #0056b3;
}
Headless components indeed focus on functionality and not the UI itself. This means they handle the core logic and behavior of an element, but they don’t dictate how it looks. They typically render basic HTML elements like buttons, divs, or lists, but leave the styling and visual presentation entirely up to the developer.
The Button Element in the Example:
In the example, the HeadlessButton
component renders a regular HTML <button>
element. This is because headless components still need to provide a basic structure for the functionality to work. The button element allows the component to capture user interaction (clicks) and trigger the defined behavior (onClick
function).
Best Practices for Developers
Headless UI components offer a powerful approach to building UIs, but their effectiveness hinges on how they’re designed and implemented. Here are some key best practices to consider when developing headless UI components, ensuring they are not only functional but also well-structured, easy to use, and maintainable by you and your fellow developers.
Focus on Core Functionality:
- Prioritize clear and concise implementation of the component’s core functionality.
- Strive for well-defined behavior that is easy to understand and use by other developers who will consume the component.
Define a Clear API:
- Design a clean and well-documented API for your headless component.
- This includes props, events, and any return values the component might have.
- Use clear prop names and consider type checking (like TypeScript) to enhance developer experience and prevent misuse.
Prioritize Accessibility:
- Build accessibility into your headless components from the ground up.
- Use appropriate ARIA attributes and semantic HTML elements to ensure your components are usable by everyone.
Write Unit Tests:
- Implement unit tests for your headless components to ensure they function as expected in isolation.
- Test different scenarios and edge cases to guarantee reliable functionality.
Consider Componentization:
- Break down complex functionality into smaller, more manageable sub-components.
- This promotes code reusability and simplifies testing.
Think About Customization:
- While headless components focus on functionality, consider offering ways for developers to customize them.
- This could involve props that control behavior or slots for injecting custom UI elements.
Provide Usage Examples:
- Include clear and concise usage examples in your component’s documentation.
- These examples should showcase how to use the component effectively in different scenarios.
Document Everything:
- Create comprehensive documentation for your headless components.
- This should include a detailed explanation of the component’s purpose, usage instructions, prop descriptions, and any accessibility considerations. Aim to make it easy for other developers to understand and integrate your component into their projects.
By following these best practices, you can develop robust, well-documented, and reusable headless UI components that empower other developers and contribute to a strong and maintainable codebase.
Conclusion:
Headless UI components offer a compelling alternative to traditional UI libraries. By separating functionality from presentation, they empower developers to build flexible, customizable, and accessible user interfaces.
Key Takeaways:
Headless UI components prioritize core functionality, allowing developers to style them according to their design system.
This separation of concerns promotes reusability, accessibility, and a lighter-weight codebase.
While requiring more development effort for styling and UX decisions, headless UI components offer greater control over the final look and feel.
The Future of Headless UI Components:
The popularity of headless UI components is on the rise. As web development continues to emphasize flexibility, accessibility, and developer experience, headless UI libraries are likely to play an increasingly important role. We can expect to see:
Continued growth and innovation in headless UI libraries, offering a wider range of components and functionalities.
Improved tooling and frameworks that streamline the development process with headless UI components.
Greater adoption of headless UI components as a standard practice for building modern web applications.
By delving into the concepts and best practices shared in this article, I trust that you’ll be equipped to harness the potential of headless UI components, empowering you to craft remarkable user interfaces for your web development endeavors.
About The Author
Deepu Balan
Hi there! I’m a web UI/UX architect. This is my online playground, my beautiful space to share my passions, my work and what I find inspiring.